Menguasai OOP di Java: Memaksimalkan Kekuatan Pemrograman Berorientasi Objek
- Deskripsi
- Materi
- Ulasan
Kursus ini dirancang untuk membantu peserta memahami dan mengimplementasikan Object-Oriented Programming (OOP) dalam Java, sebuah paradigma pemrograman yang memungkinkan pengembangan aplikasi yang lebih modular, fleksibel, dan mudah dipelihara.
Java adalah salah satu bahasa pemrograman yang paling banyak digunakan di dunia, terutama dalam pengembangan aplikasi berbasis enterprise, mobile, dan web. Dengan memahami OOP di Java, peserta akan mampu membangun kode yang lebih terstruktur, efisien, dan dapat digunakan kembali dalam proyek skala besar maupun kecil.
Dalam kursus ini, peserta akan belajar konsep dasar OOP, bagaimana menerapkannya dalam kode Java, serta bagaimana mengelola struktur kode yang baik agar mudah dikembangkan dan diperluas.
Apa yang Akan Dipelajari?
1. Pengenalan OOP dalam Java
- Konsep Object-Oriented Programming (OOP) dan perbedaannya dengan pemrograman prosedural.
- Peran Java sebagai bahasa pemrograman berbasis objek.
- Struktur dasar kelas (class) dan objek (object) dalam Java.
2. Empat Pilar OOP dalam Java
a. Enkapsulasi (Encapsulation)
- Menggunakan access modifier (private, public, protected) untuk melindungi data.
- Pembuatan metode getter dan setter untuk mengontrol akses ke atribut dalam kelas.
b. Pewarisan (Inheritance)
- Menerapkan pewarisan menggunakan extends untuk menghindari duplikasi kode.
- Konsep superclass dan subclass dalam Java.
c. Polimorfisme (Polymorphism)
- Memahami method overloading dan method overriding.
- Penerapan dynamic method dispatch untuk fleksibilitas dalam implementasi metode.
d. Abstraksi (Abstraction)
- Penggunaan abstract class dan interface untuk menyederhanakan kompleksitas kode.
- Perbedaan antara abstraksi penuh (interface) dan abstraksi sebagian (abstract class).
3. Struktur Kelas dan Objek yang Modular
- Perancangan hubungan antar objek menggunakan UML (Unified Modeling Language).
- Pembuatan constructor untuk inisialisasi objek.
- Pengelolaan static dan instance variables dalam aplikasi.
4. Manajemen dan Pemeliharaan Kode
- Prinsip SOLID dalam desain perangkat lunak berbasis OOP.
- Penggunaan Design Patterns dalam pengembangan Java.
- Penerapan modular programming untuk meningkatkan keterbacaan dan pemeliharaan kode.
5. Implementasi OOP dalam Aplikasi Java
- Pengembangan aplikasi berbasis OOP dengan menerapkan prinsip yang telah dipelajari.
- Mengelola data dan interaksi antar objek dalam aplikasi.
- Menerapkan exception handling agar aplikasi lebih stabil.
6. Pengujian dan Debugging Program
- Melakukan unit testing menggunakan JUnit.
- Teknik debugging untuk menemukan dan memperbaiki bug dalam kode.
- Menggunakan exception handling untuk menangani error dengan lebih baik.
7. Dokumentasi dan Penyajian Proyek
Â
- Menulis dokumentasi proyek yang mencakup deskripsi aplikasi, arsitektur sistem, dan cara penggunaan aplikasi.
- Menggunakan diagram UML untuk menjelaskan hubungan antar kelas.
- Mempresentasikan proyek yang telah dikembangkan.
-
1Class And Object Theory in JavaPratinjau 5.48
-
2JDK JRE JVM in JavaSorry, this lesson is currently locked. You need to complete "Class And Object Theory in Java" before accessing it.
-
3Methods in JavaSorry, this lesson is currently locked. You need to complete "JDK JRE JVM in Java" before accessing it.
-
4Complete Java Developer CourseSorry, this lesson is currently locked. You need to complete "Methods in Java" before accessing it.
-
5Method Overloading in JavaSorry, this lesson is currently locked. You need to complete "Complete Java Developer Course" before accessing it.
-
6Stack And Heap in JavaSorry, this lesson is currently locked. You need to complete "Method Overloading in Java" before accessing it.
-
7Need of an Array in JavaSorry, this lesson is currently locked. You need to complete "Stack And Heap in Java" before accessing it.
-
8Creation of Array in JavaSorry, this lesson is currently locked. You need to complete "Need of an Array in Java" before accessing it.
-
9Multi Dimensional Array in JavaSorry, this lesson is currently locked. You need to complete "Creation of Array in Java" before accessing it.
-
10jagged and 3D Array in JavaSorry, this lesson is currently locked. You need to complete "Multi Dimensional Array in Java" before accessing it.
-
11Drawbacks of Array in JavaSorry, this lesson is currently locked. You need to complete "jagged and 3D Array in Java" before accessing it.
-
12Spring Boot 3 and Spring AISorry, this lesson is currently locked. You need to complete "Drawbacks of Array in Java" before accessing it.
-
13Array of Objects in JavaSorry, this lesson is currently locked. You need to complete "Spring Boot 3 and Spring AI" before accessing it.
-
14Enhanced for Loop in JavaSorry, this lesson is currently locked. You need to complete "Array of Objects in Java" before accessing it.
-
15What is String in JavaSorry, this lesson is currently locked. You need to complete "Enhanced for Loop in Java" before accessing it.
-
16Mutable vs Immutable String in JavaSorry, this lesson is currently locked. You need to complete "What is String in Java" before accessing it.
-
17StringBuffer and StringBuilder in JavaSorry, this lesson is currently locked. You need to complete "Mutable vs Immutable String in Java" before accessing it.
-
18Static Variable in JavaSorry, this lesson is currently locked. You need to complete "StringBuffer and StringBuilder in Java" before accessing it.
-
19Static Block in javaSorry, this lesson is currently locked. You need to complete "Static Variable in Java" before accessing it.
-
20Static Method in JavaSorry, this lesson is currently locked. You need to complete "Static Block in java" before accessing it.
-
21Getters and Setters in JavaSorry, this lesson is currently locked. You need to complete "Static Method in Java" before accessing it.
-
22Encapsulation in JavaSorry, this lesson is currently locked. You need to complete "Getters and Setters in Java" before accessing it.
-
23This keyword in JavaSorry, this lesson is currently locked. You need to complete "Encapsulation in Java" before accessing it.
-
24Constructor in JavaSorry, this lesson is currently locked. You need to complete "This keyword in Java" before accessing it.
-
25Default vs Parameterized Constructor in JavaSorry, this lesson is currently locked. You need to complete "Constructor in Java" before accessing it.
-
26This and Super Method in JavaSorry, this lesson is currently locked. You need to complete "Default vs Parameterized Constructor in Java" before accessing it.
-
27Naming Convention in JavaSorry, this lesson is currently locked. You need to complete "This and Super Method in Java" before accessing it.
-
28Premium Java and spring boot CoursesSorry, this lesson is currently locked. You need to complete "Naming Convention in Java" before accessing it.
-
29Anonymous Object in javaSorry, this lesson is currently locked. You need to complete "Premium Java and spring boot Courses" before accessing it.
-
30Need of Inheritance in JavaSorry, this lesson is currently locked. You need to complete "Anonymous Object in java" before accessing it.
-
31What is Inheritance in JavaSorry, this lesson is currently locked. You need to complete "Need of Inheritance in Java" before accessing it.
-
32Single and Multilevel inheritance in javaSorry, this lesson is currently locked. You need to complete "What is Inheritance in Java" before accessing it.
-
33Multiple Inheritance in JavaSorry, this lesson is currently locked. You need to complete "Single and Multilevel inheritance in java" before accessing it.
-
34Method Overriding in JavaSorry, this lesson is currently locked. You need to complete "Multiple Inheritance in Java" before accessing it.
-
35Packages in JavaSorry, this lesson is currently locked. You need to complete "Method Overriding in Java" before accessing it.
-
36Access Modifiers in JavaSorry, this lesson is currently locked. You need to complete "Packages in Java" before accessing it.
-
37Polymorphism in JavaSorry, this lesson is currently locked. You need to complete "Access Modifiers in Java" before accessing it.
-
38Dynamic Method Dispatch in JavaSorry, this lesson is currently locked. You need to complete "Polymorphism in Java" before accessing it.
-
39Final keyword in javaSorry, this lesson is currently locked. You need to complete "Dynamic Method Dispatch in Java" before accessing it.
-
40Object Class equals toString hashcode in JavaSorry, this lesson is currently locked. You need to complete "Final keyword in java" before accessing it.
-
41Upcasting and Downcasting in JavaSorry, this lesson is currently locked. You need to complete "Object Class equals toString hashcode in Java" before accessing it.
-
42Abstract Keyword in JavaSorry, this lesson is currently locked. You need to complete "Upcasting and Downcasting in Java" before accessing it.
-
43Inner Class in JavaSorry, this lesson is currently locked. You need to complete "Abstract Keyword in Java" before accessing it.
-
44Anonymous Inner Class in JavaSorry, this lesson is currently locked. You need to complete "Inner Class in Java" before accessing it.
-
45Abstract and Anonymous Inner ClassSorry, this lesson is currently locked. You need to complete "Anonymous Inner Class in Java" before accessing it.
-
46Need of Interface in JavaSorry, this lesson is currently locked. You need to complete "Abstract and Anonymous Inner Class" before accessing it.
-
47What is Interface in JavaSorry, this lesson is currently locked. You need to complete "Need of Interface in Java" before accessing it.
-
48More on Interfaces in JavaSorry, this lesson is currently locked. You need to complete "What is Interface in Java" before accessing it.
-
49What is Enum in JavaSorry, this lesson is currently locked. You need to complete "More on Interfaces in Java" before accessing it.
-
50Enum if and Switch in JavaSorry, this lesson is currently locked. You need to complete "What is Enum in Java" before accessing it.
-
51Enum Class in JavaSorry, this lesson is currently locked. You need to complete "Enum if and Switch in Java" before accessing it.
-
52What is Annotation in JavaSorry, this lesson is currently locked. You need to complete "Enum Class in Java" before accessing it.
-
53Functional Interface New in JavaSorry, this lesson is currently locked. You need to complete "What is Annotation in Java" before accessing it.
-
54Lambda Expression in JavaSorry, this lesson is currently locked. You need to complete "Functional Interface New in Java" before accessing it.
-
55Lambda Expression with returnSorry, this lesson is currently locked. You need to complete "Lambda Expression in Java" before accessing it.
-
56Types of Interface in JavaSorry, this lesson is currently locked. You need to complete "Lambda Expression with return" before accessing it.
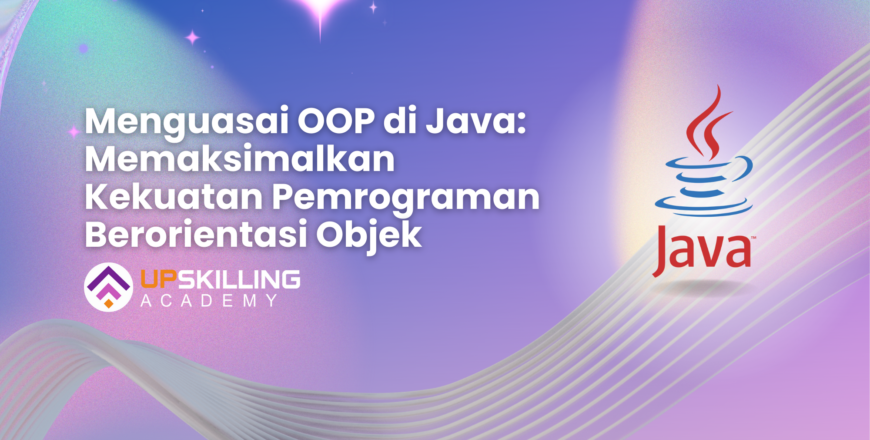
Class And Object Theory in Java
Jam Kerja
Monday | 07.00 WIB - 16.00 WIB |
Tuesday | 08.00 WIB - 15.00 WIB |
Wednesday | 06.00 WIB - 15.00 WIB |
Thursday | 07.00 WIB - 16.00 WIB |
Friday | 08.00 WIB - 15.00 WIB |
Saturday | Closed |
Sunday | Closed |